SDK & Bibliotek
Gjord för utvecklare.
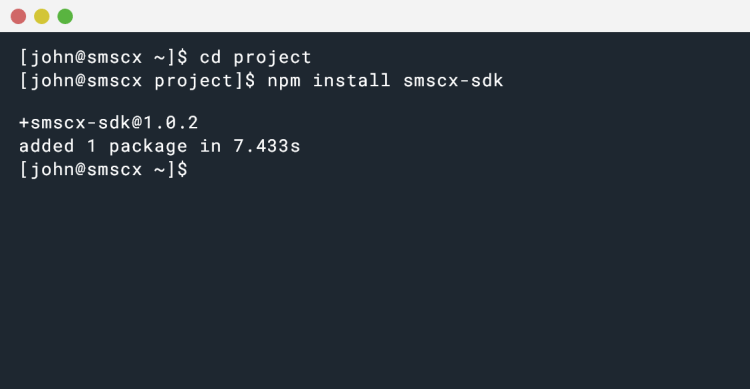
Använd våra bibliotek för en snabbare integration
Installation
The simplest way to install the library is to get it from PyPi using pip, which is a Python package manager. Just type this into the terminal:
pip install smscx-client
Requirements
Python >=3.6
After installation, import the package:
import smscx_client
Authentication
To use the library you must authenticate. SMS.CX Python Library supports the same authentication methods as those supported by the SMS Connexion API:
Handling errors
The SMS.CX Python library raises an exception for every error type. It is recommended to catch and handle exceptions.
To catch an exception, use Python’s try/except syntax. SMS Connexion provides many exception classes you can catch. Each one represents a different kind of error. When you catch an exception, you can use its class to choose a response.
General exceptions:
DuplicateIdException
- A resource with the same ID already existDuplicateValueException
- You are trying to create/update a resource that must be unique (eg. originators, group name, shortlinks, template name)InsufficientScopeException
- Your application does not have the privilege to access a resourceInvalidCredentialsException
- Unable to authenticate you based on the information provided.InvalidRequestException
- The parameters provided were invalidInvalidScopeException
- The scope requested does not existRateLimitExcedeedException
- You made too many API calls in short period of time.ResourceNotFoundException
- The ID of the requested resource was not found (eg. group, campaign, otp, shortlink, template, etc.)ApiMethodNotAllowedException
- The target resource doesn’t support this HTTP methodAccessDeniedException
- You don’t have permission to perform an action (eg. editing a template that was locked, replying to an Whatsapp after more than 24 hours passed from client reply, etc.)ServerErrorException
- Something went wrong on SMS Connexion’s side.ApiException
- Something went wrong on SMS Connexion’s side
Exceptions for methods that validate numbers or incur costs (to send SMS, add phone numbers to groups, validate number, etc.):
InsufficientBalanceException
- Your request incurs charges in excess of your existing balance.InvalidPhoneNumberException
- The phone number provided is not valid
Exceptions for methods that require network coverage (send SMS, Viber, Whatsapp):
NoCoverageException
- There is no coverage for the destination requested (these are rare)
Exceptions for Otp:
InvalidPinException
- The PIN provided does not verify with our recordsOtpAlreadyVerifiedException
- The OTP was already verifiedOtpCancelledException
- You cannot verify an OTP that was cancelledOtpActionNotAllowedException
- You cannot cancel an OTP that has non-pending status (eg. was already verified, canceled, or expired)OtpExpiredException
- You cannot verify an OTP that was expiredOtpFailedException
- The OTP verification has failed because the numbers of max attempts was reached
Exceptions for Viber/Whatsapp:
ChannelNotActiveException
- Channel is not active. You need to register Viber and Whatsapp by contacting usTemplateNotApprovedException
- Template for sending Viber or Whatsapp is not approved
Examples
The library needs to be configured with your account's Application ID & Secret or an API Key which are available in your SMS.CX Dashboard.
Table of contents examples Python
Get an access token
import time
import smscx_client
from smscx_client.api import oauth_api
from smscx_client.model.oauth_token_response import OauthTokenResponse
from pprint import pprint
configuration = smscx_client.Configuration(
username = "YOUR_APPLICATION_ID",
password = "YOUR_APPLICATION_SECRET"
)
# Create an instance of the API class
api_instance = oauth_api.OauthApi(
smscx_client.ApiClient(configuration)
)
# A list of space-delimited, case-sensitive strings
# If left empty or ommited, the issued access token will be granted with all scopes (full privileges) (optional)
scope = "sms groups templates numbers shortlinks attachments"
try:
# Get access token
api_response = api_instance.get_access_token(scope=scope)
pprint(api_response)
access_token = api_response.access_token
expires_in = api_response.expires_in
except smscx_client.InvalidRequestException as e:
# Code for invalid request # noqa: E501
except smscx_client.InvalidCredentialsException as e:
# Code for invalid credentials # noqa: E501
except smscx_client.InvalidScopeException as e:
# Code for invalid scope # noqa: E501
except smscx_client.ApiException as e:
print("Exception when calling OauthApi->get_access_token: %s\n" % e)
error_response = json.loads(e.body)
error_type = error_response['error']['type']
error_code = error_response['error']['code']
error_msg = error_response['error']['message']
http_code = e.status
Up to Table of Contents
Send single SMS
import time
import smscx_client
from smscx_client.api import sms_api
from smscx_client.model.send_sms_message_request import SendSmsMessageRequest
from smscx_client.model.send_sms_message_response import SendSmsMessageResponse
from pprint import pprint
configuration = smscx_client.Configuration(
# Use authentication via API Key
api_key = "YOUR_API_KEY",
# Uncomment to use authentication via Access Token
# access_token = "YOUR_ACCESS_TOKEN",
)
# Create an instance of the API class
api_instance = sms_api.SmsApi(
smscx_client.ApiClient(configuration)
)
send_sms_message_request = SendSmsMessageRequest(
to=["+31612469xxx"],
_from = "InfoText",
text = "Your confirmation code is 15997",
)
try:
# Send single SMS
api_response = api_instance.send_sms(send_sms_message_request)
pprint(api_response)
# api_response.info.total_cost
for item in api_response.data:
# item.msg_id
except smscx_client.NoCoverageException as e:
# Code for No coverage
except smscx_client.InvalidRequestException as e:
# Code for invalid request
except smscx_client.InvalidPhoneNumberException as e:
# Code for invalid phone number
except smscx_client.InsufficientBalanceException as e:
# Code for insufficient balance
except smscx_client.ApiException as e:
print("Exception when calling SmsApi->send_sms: %s\n" % e)
error_response = json.loads(e.body)
error_response = json.loads(e.body)
error_type = error_response['error']['type']
error_code = error_response['error']['code']
error_msg = error_response['error']['message']
http_code = e.status
Up to Table of Contents
Send bulk SMS (up to 25.000 phone numbers)
An array with phone numbers in E.164 format must be supplied.
import time
import smscx_client
from smscx_client.api import sms_api
from smscx_client.model.send_sms_message_request import SendSmsMessageRequest
from smscx_client.model.send_sms_message_response import SendSmsMessageResponse
from smscx_client.model.transliteration import Transliteration
from pprint import pprint
configuration = smscx_client.Configuration(
# Use authentication via API Key
api_key = "YOUR_API_KEY",
# Uncomment to use authentication via Access Token
# access_token = "YOUR_ACCESS_TOKEN",
)
# Create an instance of the API class
api_instance = sms_api.SmsApi(
smscx_client.ApiClient(configuration)
)
send_sms_message_request = SendSmsMessageRequest(
to=[
"+31612469xxx",
"+4474005085xx",
"+49151238353xx",
"+417811126xx",
"+3519123350xx",
"+4206016090xx",
"+359483059xx",
"+336127904xx",
"+436645595xx",
"+3519121385xx",
"+3069125917xx",
],
_from="InfoText",
text="Redeem this voucher and you will get 30% discount off all Summer Fashion {{optoutLink}}",
idempotency_id="bf325375-e030-fccb-a009-17317c574773",
transliteration=Transliteration(
alphabet="NONE",
remove_emojis=False,
),
)
try:
# Send SMS
api_response = api_instance.send_sms(send_sms_message_request)
pprint(api_response)
# api_response.info.total_cost
for item in api_response.data:
# item.msg_id
except smscx_client.NoCoverageException as e:
# Code for No coverage
except smscx_client.InvalidRequestException as e:
# Code for invalid request
except smscx_client.InvalidPhoneNumberException as e:
# Code for invalid phone number
except smscx_client.InsufficientBalanceException as e:
# Code for insufficient balance
except smscx_client.ApiException as e:
print("Exception when calling SmsApi->send_sms: %s\n" % e)
error_response = json.loads(e.body)
error_type = error_response['error']['type']
error_code = error_response['error']['code']
error_msg = error_response['error']['message']
http_code = e.status
Up to Table of Contents
Create new OTP request
import time
import smscx_client
from smscx_client.api import otp_api
from smscx_client.model.new_otp_request import NewOtpRequest
from pprint import pprint
configuration = smscx_client.Configuration(
# Use authentication via API Key
api_key = "YOUR_API_KEY",
# Uncomment to use authentication via Access Token
# access_token = "YOUR_ACCESS_TOKEN",
)
# Create an instance of the API class
api_instance = otp_api.OtpApi(
smscx_client.ApiClient(configuration)
)
new_otp_request = NewOtpRequest(
phone_number="+336122464xx",
country_iso="FR",
_from="InfoText",
template="Your verification code is {{pin}}",
track_id="bf325375-e030-fccb-a009-17317c574773",
ttl=180,
max_attempts=4,
pin_type="numbers",
pin_length=4,
otp_callback_url="https://webhook/receive-otp-status-updates",
)
try:
# New OTP
api_response = api_instance.new_otp(new_otp_request)
pprint(api_response)
# api_response.otp_id
# api_response.track_id
# api_response.phone_number
# api_response.country_iso
# api_response.status
# api_response.cost
# api_response.parts
# api_response.max_attempts
# api_response.attempts
# api_response.ttl
# api_response.otp_callback_url
# api_response.date_created
# api_response.date_expires
except smscx_client.InvalidRequestException as e:
# Code for invalid request
except smscx_client.InvalidPhoneNumberException as e:
# Code for invalid phone number
except smscx_client.InsufficientBalanceException as e:
# Code for insufficient balance
except smscx_client.ApiException as e:
print("Exception when calling OtpApi->new_otp: %s\n" % e)
Up to Table of Contents
Verify OTP
import time
import smscx_client
from smscx_client.api import otp_api
from smscx_client.model.verify_pin_request import VerifyPinRequest
from pprint import pprint
configuration = smscx_client.Configuration(
# Use authentication via API Key
api_key = "YOUR_API_KEY",
# Uncomment to use authentication via Access Token
# access_token = "YOUR_ACCESS_TOKEN",
)
# Create an instance of the API class
api_instance = otp_api.OtpApi(
smscx_client.ApiClient(configuration)
)
otp_id = "a17fb13d-f4ac-4d93-9439-ef41ab8de390"
pin = "1544"
try:
# Verify OTP
api_response = api_instance.verify_otp(otp_id, pin)
pprint(api_response)
if api_response.status = 'VERIFIED'
# PIN successfully verified
pprint('verified')
except smscx_client.InvalidPinException as e:
# Code for Invalid PIN
except smscx_client.OtpAlreadyVerifiedException as e:
# Code for OTP already verified
except smscx_client.OtpCancelledException as e:
# Code for OTP cancelled
except smscx_client.OtpExpiredException as e:
# Code for OTP expired
except smscx_client.OtpFailedException as e:
# Code for OTP failed
except smscx_client.ApiException as e:
print("Exception when calling OtpApi->verify_otp: %s\n" % e)
Up to Table of Contents
Validate phone numbers in bulk (up to 40.000 phone numbers)
With phone number validation you can check if a number is valid by checking the network prefix for each country. An array with phone numbers in E.164 (eg. +316124692xx) or national (eg. 06124692xx) format must be supplied.
import time
import smscx_client
from smscx_client.api import numbers_api
from smscx_client.model.numbers_validate_request import NumbersValidateRequest
from pprint import pprint
configuration = smscx_client.Configuration(
# Use authentication via API Key
api_key = "YOUR_API_KEY",
# Uncomment to use authentication via Access Token
# access_token = "YOUR_ACCESS_TOKEN",
)
# Create an instance of the API class
api_instance = numbers_api.NumbersApi(
smscx_client.ApiClient(configuration)
)
numbers_validate_request = NumbersValidateRequest(
phone_numbers=[
"+336124241xx",
"+336123",
"+336129564xx",
"+420604558xx",
"+336123345xx",
"+4474006505xx",
"+49151237483xx",
"+49151286205xx",
"+4206018488xx",
"+49151232142xx",
"+3934237620xx",
"+336129564xx",
# National format if parameter country_iso is used
"06124241xx",
],
# country_iso="FR",
) # NumbersValidateRequest |
try:
# Validate numbers in bulk
api_response = api_instance.validate_numbers(numbers_validate_request)
pprint(api_response)
# api_response.info.total_phone_numbers
# api_response.info.total_valid
# api_response.info.total_invalid
for item in api_response.data:
# item.phone_number
# item.country_iso
# item.network_operator
# item.timezone
# item.invalid
except smscx_client.InvalidRequestException as e:
# Code for invalid request
except smscx_client.ApiException as e:
print("Exception when calling NumbersApi->validate_numbers: %s\n" % e)
Up to Table of Contents
Lookup phone numbers in bulk (up to 40.000 phone numbers)
With phone number lookup you can check if a number is active or absent in the network, if the number is ported and the original and existing network name. An array with phone numbers in E.164 (eg. +316124694xx) or national (eg. 06124694xx) format must be supplied.
import time
import smscx_client
from smscx_client.api import numbers_api
from smscx_client.model.numbers_lookup_request import NumbersLookupRequest
from pprint import pprint
configuration = smscx_client.Configuration(
# Use authentication via API Key
api_key = "YOUR_API_KEY",
# Uncomment to use authentication via Access Token
# access_token = "YOUR_ACCESS_TOKEN",
)
# Create an instance of the API class
api_instance = numbers_api.NumbersApi(
smscx_client.ApiClient(configuration)
)
numbers_lookup_request = NumbersLookupRequest(
phone_numbers=[
"+336124241xx",
"+336123",
"+336129564xx",
"+420604558xx",
"+336123345xx",
"+4474006505xx",
"+49151237483xx",
"+49151286205xx",
"+4206018488xx",
"+49151232142xx",
"+3934237620xx",
"+336129564xx",
# National format if parameter country_iso is used
"06124241xx",
],
country_iso="FR",
lookup_callback_url="https://my-callback-url/receive-lookup-details",
)
try:
# Lookup numbers in bulk
api_response = api_instance.lookup_numbers(numbers_lookup_request)
pprint(api_response)
# api_response.info.lookup_bulk_id
# api_response.info.total_phone_numbers
# api_response.info.total_valid
# api_response.info.total_invalid
# api_response.info.cost
# api_response.info.invalid
except smscx_client.NoCoverageException as e:
# Code for No coverage
except smscx_client.InvalidRequestException as e:
# Code for invalid request
except smscx_client.InvalidPhoneNumberException as e:
# Code for invalid phone number
except smscx_client.InsufficientBalanceException as e:
# Code for insufficient balance
except smscx_client.ApiException as e:
print("Exception when calling NumbersApi->lookup_numbers: %s\n" % e)
Up to Table of Contents
Get delivery report for a sent SMS campaign
import time
import smscx_client
from smscx_client.api import reports_api
from pprint import pprint
configuration = smscx_client.Configuration(
# Use authentication via API Key
api_key = "YOUR_API_KEY",
# Uncomment to use authentication via Access Token
# access_token = "YOUR_ACCESS_TOKEN",
)
# Create an instance of the API class
api_instance = reports_api.ReportsApi(
smscx_client.ApiClient(configuration)
)
campaign_id = "4baf0298-0c21-4df1-a60a-6e3476e95e0b" # str | Identifier of a sent campaign
try:
# Get campaign report
api_response = api_instance.get_campaign_report(campaign_id)
pprint(api_response)
# api_response.info.campaign_id
# api_response.info.campaign_name
# api_response.info.total_phone_numbers
# api_response.info.total_cost
# api_response.info.total_parts
# api_response.info.channel
for item in api_response.data:
# item.msg_id
# item.status
# item.status_code
# item.error_code
# item.in_quiet_hours
# item.created_at
# item.updated_at
# item.scheduled_at
# item.cost
# item.to
# item._from
# item.source
# item.channel
# item.text
# item.text_analysis
except smscx_client.ResourceNotFoundException as e:
# Code for campaign ID not found
except smscx_client.InvalidRequestException as e:
# Code for invalid request
except smscx_client.ApiException as e:
print("Exception when calling ReportsApi->get_campaign_report: %s\n" % e)
Up to Table of Contents
Rent a phone number
import time
import smscx_client
from smscx_client.api import numbers_api
from smscx_client.model.rent_number_request import RentNumberRequest
from pprint import pprint
configuration = smscx_client.Configuration(
# Use authentication via API Key
api_key = "YOUR_API_KEY",
# Uncomment to use authentication via Access Token
# access_token = "YOUR_ACCESS_TOKEN",
)
# Create an instance of the API class
api_instance = numbers_api.NumbersApi(
smscx_client.ApiClient(configuration)
)
rent_number_request = RentNumberRequest(
number_id="bf325375-e030-fccb-a009-17317c574773",
rent_period=30,
auto_renew=False,
callback_url="https://webhook/receive-inbound-sms/",
)
try:
# Rent phone number
api_response = api_instance.rent_number(rent_number_request)
pprint(api_response)
# api_response.info.rent_id
# api_response.info.number_id
# api_response.info.phone_number
# api_response.info.country_iso
# api_response.info.rent_start
# api_response.info.rent_end
# api_response.info.rent_cost
# api_response.info.setup_cost
# api_response.info.auto_renew
# api_response.info.approved
# api_response.info.callback_url
# api_response.info.datetime
except smscx_client.InvalidRequestException as e:
# Code for invalid request
except smscx_client.ResourceNotFoundException as e:
# Code for Number ID not found
except smscx_client.AccessDeniedException as e:
# Code for Number was already rented by someone else
except smscx_client.InsufficientBalanceException as e:
# Code for Insufficient balance
except smscx_client.ApiException as e:
print("Exception when calling NumbersApi->rent_number: %s\n" % e)
Up to Table of Contents
Get inbound SMS
Manually check for the received SMS on a rented phone number. This method to get the list of received SMS is an alternative to the webhook method, in which the details of the inbound SMS are sent to a specified callback URL.
import time
import smscx_client
from smscx_client.api import numbers_api
from pprint import pprint
configuration = smscx_client.Configuration(
# Use authentication via API Key
api_key = "YOUR_API_KEY",
# Uncomment to use authentication via Access Token
# access_token = "YOUR_ACCESS_TOKEN",
)
# Create an instance of the API class
api_instance = numbers_api.NumbersApi(
smscx_client.ApiClient(configuration)
)
# Identifier of the rental operation
rent_id = "471ddea7-930c-49e8-8e99-2683834dd92e"
try:
# Get inbound SMS from rented number
api_response = api_instance.get_inbound_sms(rent_id)
pprint(api_response)
# api_response.info.rent_id
# api_response.info.phone_number
# api_response.info.country_iso
for item in api_response.data:
# item.msg_id
# item._from
# item.country_iso
# item.to
# item.text
# item.cost
# item.received_at
except smscx_client.InvalidRequestException as e:
# Code for invalid request
except smscx_client.ResourceNotFoundException as e:
# Code for Rent ID not found
except smscx_client.ApiException as e:
print("Exception when calling NumbersApi->get_inbound_sms: %s\n" % e)
Up to Table of Contents
Get account balance
import time
import smscx_client
from smscx_client.api import account_api
from smscx_client.model.account_balance import AccountBalance
from pprint import pprint
configuration = smscx_client.Configuration(
# Use authentication via API Key
api_key = "YOUR_API_KEY",
# Uncomment to use authentication via Access Token
# access_token = "YOUR_ACCESS_TOKEN",
)
# Create an instance of the API class
api_instance = account_api.AccountApi(
smscx_client.ApiClient(configuration)
)
try:
# Get account balance
api_response = api_instance.get_account_balance()
pprint(api_response)
# api_response.balance
# api_response.currency
# api_response.billing
except smscx_client.ApiException as e:
print("Exception when calling AccountApi->get_account_balance: %s\n" % e)
Up to Table of Contents
Installation
The install the library we recommend to use Composer, which will also install the required dependencies:
composer require smscx/smscx-api-client
To use the library, use Composer's autoload:
require_once('vendor/autoload.php');
Dependencies
The library require the following extensions in order to work properly:
- ext-curl
- ext-json
- ext-mbstring
- Guzzle (PHP HTTP client that makes it easy to send HTTP requests )
- GuzzleHttp\Psr7 (PSR-7 interface for requests, responses, and streams)
Authentication
To use the library you must authenticate. SMS.CX PHP Library supports the authentication methods supported by the SMS Connexion API:
Handling errors
The SMS.CX PHP library raises an exception for every error type. It is recommended to catch and handle exceptions.
To catch an exception, use PHP’s try/catch syntax. SMS Connexion provides many exception classes you can catch. Each one represents a different kind of error. When you catch an exception, you can use its class to choose a response.
General exceptions:
DuplicateIdException
- A resource with the same ID already existDuplicateValueException
- You are trying to create/update a resource that must be unique (eg. originators, group name, shortlinks, template name)InsufficientScopeException
- Your application does not have the privilege to access a resourceInvalidCredentialsException
- Unable to authenticate you based on the information provided.InvalidRequestException
- The parameters provided were invalidInvalidScopeException
- The scope requested does not existRateLimitExcedeedException
- You made too many API calls in short period of time.ResourceNotFoundException
- The ID of the requested resource was not found (eg. group, campaign, otp, shortlink, template, etc.)ApiMethodNotAllowedException
- The target resource doesn’t support this HTTP methodAccessDeniedException
- You don’t have permission to perform an action (eg. editing a template that was locked, replying to an Whatsapp after more than 24 hours passed from client reply, etc.)ServerErrorException
- Something went wrong on SMS Connexion’s side.ApiException
- Something went wrong on SMS Connexion’s side
Exceptions for methods that validate numbers or incur costs (to send SMS, add phone numbers to groups, validate number, etc.):
InsufficientBalanceException
- Your request incurs charges in excess of your existing balance.InvalidPhoneNumberException
- The phone number provided is not valid
Exceptions for methods that require network coverage (send SMS, Viber, Whatsapp):
NoCoverageException
- There is no coverage for the destination requested (these are rare)
Exceptions for Otp:
InvalidPinException
- The PIN provided does not verify with our recordsOtpAlreadyVerifiedException
- The OTP was already verifiedOtpCancelledException
- You cannot verify an OTP that was cancelledOtpActionNotAllowedException
- You cannot cancel an OTP that has non-pending status (eg. was already verified, canceled, or expired)OtpExpiredException
- You cannot verify an OTP that was expiredOtpFailedException
- The OTP verification has failed because the numbers of max attempts was reached
Exceptions for Viber/Whatsapp:
ChannelNotActiveException
- Channel is not active. You need to register Viber and Whatsapp by contacting usTemplateNotApprovedException
- Template for sending Viber or Whatsapp is not approved
Examples
The library needs to be configured with your account's Application ID & Secret or an API Key which are available in your SMS.CX Dashboard.
Table of contents examples PHP
Get an access token
<?php
require_once(__DIR__ . '/vendor/autoload.php');
$config = Smscx\Client\Configuration::getDefaultConfiguration()
->setApplicationId('YOUR_APPLICATION_ID')
->setApplicationSecret('YOUR_APPLICATION_SECRET');
$smscx = new Smscx\Client\Api\OauthApi(
new GuzzleHttp\Client(),
$config
);
// A list of space-delimited, case-sensitive strings.
// If left empty or ommited, the issued access token will be granted with all scopes (full privileges)
$scopes = 'sms groups templates numbers reports originators viber whatsapp otp';
try {
$result = $smscx->getAccessToken($scopes);
print_r($result);
//$result->getAccessToken();
//$result->getExpiresIn();
//$result->getTokenType();
//$result->getScope();
} catch (InvalidArgumentException $e) {
//Code for Invalid argument provided
} catch (Smscx\Client\Exception\InvalidRequestException $e) {
//Code for Invalid request
} catch (Smscx\Client\Exception\InvalidCredentialsException $e) {
//Code for Invalid credentials
} catch (Smscx\Client\Exception\InvalidScopeException $e) {
//Code for Invalid scope
} catch (Smscx\Client\Exception\ApiException $e) {
echo 'Exception when calling OauthApi->getAccessToken: ', $e->getMessage(), PHP_EOL;
}
Up to Table of Contents
Send single SMS
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Use authentication via API Key
$config = Smscx\Client\Configuration::getDefaultConfiguration()->setApiKey('YOUR_API_KEY');
// OR
// Use authentication via Access Token
// $config = Smscx\Client\Configuration::getDefaultConfiguration()->setAccessToken('YOUR_ACCESS_TOKEN');
$smscx = new Smscx\Client\Api\SmsApi(
new GuzzleHttp\Client(),
$config
);
$send_sms_message_request = [
'to' => '+316124694xx',
'from' => 'InfoText',
'text' => 'Your confirmation code is 15997',
];
try {
$result = $smscx->sendSms($send_sms_message_request);
print_r($result);
// $result->getInfo()->getCampaignId();
// $result->getInfo()->getTotalPhoneNumbers();
// $result->getInfo()->getTotalValid();
// $result->getInfo()->getTotalInvalid();
// $result->getInfo()->getTotalCost();
// $result->getInfo()->getTotalParts();
// $result->getInfo()->getPhoneNumbersByCountry();
// $result->getInfo()->getTransliterationAnalysis();
// $result->getInfo()->getTotalInQuietHours();
// $result->getInfo()->getInvalid();
// $result->getInfo()->getScheduled();
foreach ( $result->getData() as $k => $v ) {
// $v->getMsgId();
// $v->getTrackData();
// $v->getStatus();
// $v->getStatusCode();
// $v->getErrorCode();
// $v->getCreatedAt();
// $v->getScheduledAt();
// $v->getInQuietHours();
// $v->getCost();
// $v->getTo();
// $v->getCountryIso();
// $v->getFrom();
// $v->getText();
// $v->getParts();
// $v->getTextAnalysis();
}
} catch (InvalidArgumentException $e) {
//Code for Invalid argument provided
} catch (Smscx\Client\Exception\NoCoverageException $e) {
//Code for No coverage
} catch (Smscx\Client\Exception\InvalidRequestException $e) {
//Code for Invalid request
} catch (Smscx\Client\Exception\InvalidPhoneNumberException $e) {
//Code for Invalid phone number
} catch (Smscx\Client\Exception\InsufficientBalanceException $e) {
//Code for Insufficient balance
} catch (Smscx\Client\ApiException $e) {
echo 'Exception when calling SmsApi->sendSms: ', $e->getMessage(), PHP_EOL;
// $httpCode = $e->getCode(); # HTTP code: 400, 401, 403, 429, 500, etc
// $errorType = $e->getResponseObject()->getError()->getType(); # Error type: invalid_param, insufficient_credit, rate_limit, etc
// $errorMessage = $e->getResponseObject()->getError()->getMessage(); # Short description of the error
// $errorCode = $e->getResponseObject()->getError()->getCode(); # API internal code: 1208, 1101, 1221, etc
}
Up to Table of Contents
Send bulk SMS (up to 25.000 phone numbers)
An array with phone numbers in E.164 format must be supplied.
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Use authentication via API Key
$config = Smscx\Client\Configuration::getDefaultConfiguration()->setApiKey('YOUR_API_KEY');
// OR
// Use authentication via Access Token
// $config = Smscx\Client\Configuration::getDefaultConfiguration()->setAccessToken('YOUR_ACCESS_TOKEN');
$smscx = new Smscx\Client\Api\SmsApi(
new GuzzleHttp\Client(),
$config
);
$send_sms_message_request = [
//Max 25.000 phone numbers
'to' => [
'+316124693xx',
'+4474005085xx',
'+49151238353xx',
'+417811126xx',
'+3519123350xx',
'+4206016090xx',
'+359483059xx',
'+336127904xx',
'+436645595xx',
'+3519121385xx',
'+3069125917xx',
],
'from' => 'InfoText',
'text' => 'Redeem this voucher and you will get 30% discount off all Summer Fashion {{optoutLink}}',
'allowInvalid' => true,
'transliteration' => [
'alphabet' => 'NON_GSM',
'removeEmojis' => true,
],
'idempotencyId' => '854cd53f-d77d-4aa8-9bd9-fbf720f3332d',
];
try {
$result = $smscx->sendSms($send_sms_message_request);
print_r($result);
// $result->getInfo()->getCampaignId();
// $result->getInfo()->getTotalPhoneNumbers();
// $result->getInfo()->getTotalValid();
// $result->getInfo()->getTotalInvalid();
// $result->getInfo()->getTotalCost();
// $result->getInfo()->getTotalParts();
// $result->getInfo()->getDuplicatesRemoved();
// $result->getInfo()->getDlrCallbackUrl();
// $result->getInfo()->getPhoneNumbersByCountry();
// $result->getInfo()->getGroups();
// $result->getInfo()->getTransliterationAnalysis();
// $result->getInfo()->getTotalInQuietHours();
// $result->getInfo()->getInvalid();
// $result->getInfo()->getScheduled();
foreach ( $result->getData() as $k => $v ) {
// $v->getMsgId();
// $v->getTrackData();
// $v->getStatus();
// $v->getStatusCode();
// $v->getErrorCode();
// $v->getCreatedAt();
// $v->getScheduledAt();
// $v->getInQuietHours();
// $v->getCost();
// $v->getTo();
// $v->getCountryIso();
// $v->getFrom();
// $v->getText();
// $v->getParts();
// $v->getTextAnalysis();
}
} catch (InvalidArgumentException $e) {
//Code for Invalid argument provided
} catch (Smscx\Client\Exception\NoCoverageException $e) {
//Code for No coverage
} catch (Smscx\Client\Exception\InvalidRequestException $e) {
//Code for Invalid request
} catch (Smscx\Client\Exception\InsufficientBalanceException $e) {
//Code for Insufficient balance
} catch (Smscx\Client\ApiException $e) {
echo 'Exception when calling SmsApi->sendSms: ', $e->getMessage(), PHP_EOL;
// $httpCode = $e->getCode(); # HTTP code: 400, 401, 403, 429, 500, etc
// $errorType = $e->getResponseObject()->getError()->getType(); # Error type: invalid_param, insufficient_credit, rate_limit, etc
// $errorMessage = $e->getResponseObject()->getError()->getMessage(); # Short description of the error
// $errorCode = $e->getResponseObject()->getError()->getCode(); # API internal code: 1208, 1101, 1221, etc
}
Up to Table of Contents
Create new OTP request
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Use authentication via API Key
$config = Smscx\Client\Configuration::getDefaultConfiguration()->setApiKey('YOUR_API_KEY');
// OR
// Use authentication via Access Token
// $config = Smscx\Client\Configuration::getDefaultConfiguration()->setAccessToken('YOUR_ACCESS_TOKEN');
$smscx = new Smscx\Client\Api\OtpApi(
new GuzzleHttp\Client(),
$config
);
//Model: \Smscx\Client\Model\NewOtpRequest
$new_otp_request = [
'phoneNumber' => '+336124241xx',
// Optional parameters
//'countryIso' => 'FR',
//'from' => 'Verify',
//'template' => 'Verification code: {{pin}}',
//'trackId' => '3b90b6d3-4fd6-40d8-9b2d-310cb50f201d',
//'ttl' => '500',
//'maxAttempts' => '5',
//'pinType' => 'numbers',
//'pinLength' => '5',
//'otpCallbackUrl' => 'https://callback-url/receive-otp-status',
];
try {
$result = $smscx->newOtp($new_otp_request);
print_r($result);
// $result->getOtpId();
// $result->getTrackId();
// $result->getPhoneNumber();
// $result->getCountryIso();
// $result->getStatus();
// $result->getCost();
// $result->getParts();
// $result->getMaxAttempts();
// $result->getAttempts();
// $result->getTtl();
// $result->getOtpCallbackUrl();
// $result->getDateCreated();
// $result->getDateExpires();
} catch (InvalidArgumentException $e) {
//Code for Invalid argument provided
} catch (Smscx\Client\Exception\InvalidPhoneNumberException $e) {
//Code for Invalid phone number
} catch (Smscx\Client\Exception\InsufficientBalanceException $e) {
//Code for Insufficient balance
} catch (Smscx\Client\Exception\InvalidRequestException $e) {
//Code for Invalid request
} catch (Smscx\Client\ApiException $e) {
echo 'Exception when calling OtpApi->newOtp: ', $e->getMessage(), PHP_EOL;
}
Up to Table of Contents
Verify OTP
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Use authentication via API Key
$config = Smscx\Client\Configuration::getDefaultConfiguration()->setApiKey('YOUR_API_KEY');
// OR
// Use authentication via Access Token
// $config = Smscx\Client\Configuration::getDefaultConfiguration()->setAccessToken('YOUR_ACCESS_TOKEN');
$smscx = new Smscx\Client\Api\OtpApi(
new GuzzleHttp\Client(),
$config
);
$otp_id = 'a17fb13d-f4ac-4d93-9439-ef41ab8de390';
$pin = '25414';
try {
$result = $smscx->verifyOtp($otp_id, $pin);
print_r($result);
if ( $result->getStatus() == 'VERIFIED') {
//Successfully verified
}
} catch (InvalidArgumentException $e) {
//Code for Invalid argument provided
} catch (Smscx\Client\Exception\InvalidPinException $e) {
// Code for Invalid PIN
} catch (Smscx\Client\Exception\OtpAlreadyVerifiedException $e) {
// Code for OTP already verified
} catch (Smscx\Client\Exception\OtpCancelledException $e) {
// Code for OTP cancelled
} catch (Smscx\Client\Exception\OtpExpiredException $e) {
// Code for OTP expired
} catch (Smscx\Client\Exception\OtpFailedException $e) {
// Code for OTP failed
} catch (Smscx\Client\Exception\ApiException $e) {
echo 'Exception when calling OtpApi->verifyOtp: ', $e->getMessage(), PHP_EOL;
}
Up to Table of Contents
Validate phone numbers in bulk (up to 40.000 phone numbers)
With phone number validation you can check if a number is valid by checking the network prefix for each country. An array with phone numbers in E.164 (eg. +316124692xx) or national (eg. 06124692xx) format must be supplied.
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Use authentication via API Key
$config = Smscx\Configuration::getDefaultConfiguration()->setApiKey('YOUR_API_KEY');
// OR
// Use authentication via Access Token
// $config = Smscx\Configuration::getDefaultConfiguration()->setAccessToken('YOUR_ACCESS_TOKEN');
$smscx = new Smscx\Client\Api\NumbersApi(
new GuzzleHttp\Client(),
$config
);
$numbers_validate_request = [
'phoneNumbers' => [
'+33612424105',
'+33612246450',
'+336123',
'+33612956402',
'+33612334525',
'+447400650588',
'+4915123748358',
'+4915128620584',
'+420601848808',
'+420601302207',
'+420204532112',
// National format if parameter countryIso is used
//'06124241xx',
],
//'countryIso' => 'FR',
];
try {
$result = $smscx->validateNumbers($numbers_validate_request);
print_r($result);
// $result->getInfo()->getTotalPhoneNumbers();
// $result->getInfo()->getTotalValid();
// $result->getInfo()->getTotalInvalid();
foreach ($result->getData() as $k => $v) {
// $v->getPhoneNumber();
// $v->getCountryIso();
// $v->getNetworkOperator();
// $v->getTimezone();
// $v->getInvalid(); # true or false
}
} catch (InvalidArgumentException $e) {
//Code for Invalid argument provided
} catch (Smscx\Client\Exception\InvalidRequestException $e) {
//Code for Invalid request
} catch (Smscx\Client\Exception\ApiException $e) {
echo 'Exception when calling NumbersApi->validateNumbers: ', $e->getMessage(), PHP_EOL;
}
Up to Table of Contents
Lookup phone numbers in bulk (up to 40.000 phone numbers)
With phone number lookup you can check if a number is active or absent in the network, if the number is ported and the original and existing network name. An array with phone numbers in E.164 (eg. +316124694xx) or national (eg. 06124694xx) format must be supplied.
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Use authentication via API Key
$config = Smscx\Configuration::getDefaultConfiguration()->setApiKey('YOUR_API_KEY');
// OR
// Use authentication via Access Token
// $config = Smscx\Configuration::getDefaultConfiguration()->setAccessToken('YOUR_ACCESS_TOKEN');
$smscx = new Smscx\Client\Api\NumbersApi(
new GuzzleHttp\Client(),
$config
);
$numbers_lookup_request = [
'phoneNumbers' => [
'+336124241xx',
'+336123',
'+336129564xx',
'+336124241xx',
'+420604558xx',
'+336129564xx',
'+336123345xx',
'+4474006505xx',
'+49151237483xx',
'+49151286205xx',
'+4206018488xx',
'+49151232142xx',
'+3934237620xx'
// National format if parameter countryIso is used
//'06124241xx',
],
//'countryIso' => 'FR',
'lookupCallbackUrl' => 'https://my-callback-url/receive-lookup-details',
];
try {
$result = $smscx->lookupNumbers($numbers_lookup_request);
print_r($result);
// $result->getInfo()->getLookupBulkId();
// $result->getInfo()->getTotalPhoneNumbers();
// $result->getInfo()->getTotalValid();
// $result->getInfo()->getTotalInvalid();
// $result->getInfo()->getCost();
// $result->getInfo()->getInvalid();
} catch (InvalidArgumentException $e) {
//Code for Invalid argument provided
} catch (Smscx\Client\Exception\InvalidPhoneNumberException $e) {
//Code for Invalid phone number
} catch (Smscx\Client\Exception\InvalidRequestException $e) {
//Code for Invalid request
} catch (Smscx\Client\Exception\NoCoverageException $e) {
//Code for No coverage
} catch (Smscx\Client\Exception\InsufficientBalanceException $e) {
//Code for Insufficient balance
} catch (Smscx\Client\Exception\ApiException $e) {
echo 'Exception when calling NumbersApi->lookupNumbers: ', $e->getMessage(), PHP_EOL;
}
Up to Table of Contents
Get delivery report for a sent SMS campaign
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Use authentication via API Key
$config = Smscx\Configuration::getDefaultConfiguration()->setApiKey('YOUR_API_KEY');
// OR
// Use authentication via Access Token
// $config = Smscx\Configuration::getDefaultConfiguration()->setAccessToken('YOUR_ACCESS_TOKEN');
$smscx = new Smscx\Client\Api\ReportsApi(
new GuzzleHttp\Client(),
$config
);
// Identifier of a sent SMS campaign
$campaign_id = '4baf0298-0c21-4df1-a60a-6e3476e95e0b';
try {
$result = $smscx->getCampaignReport($campaign_id);
print_r($result);
// $result->getInfo()->getCampaignId();
// $result->getInfo()->getCampaignName();
// $result->getInfo()->getTotalPhoneNumbers();
// $result->getInfo()->getTotalCost();
// $result->getInfo()->getTotalParts();
// $result->getInfo()->getChannel();
foreach ( $result->getData() as $k => $v ) {
// $v->getMsgId();
// $v->getStatus();
// $v->getStatusCode();
// $v->getErrorCode();
// $v->getInQuietHours();
// $v->getCreatedAt();
// $v->getUpdatedAt();
// $v->getScheduledAt();
// $v->getCost();
// $v->getTo();
// $v->getCountryIso();
// $v->getFrom();
// $v->getSource();
// $v->getChannel();
// $v->getText();
// $v->getTextAnalysis();
}
} catch (InvalidArgumentException $e) {
//Code for Invalid argument provided
} catch (Smscx\Client\Exception\ResourceNotFoundException $e) {
//Campaign ID not found
} catch (Smscx\Client\Exception\InvalidRequestException $e) {
//Code for Invalid request
} catch (Smscx\Client\Exception\ApiException $e) {
echo 'Exception when calling ReportsApi->getCampaignReport: ', $e->getMessage(), PHP_EOL;
}
Up to Table of Contents
Rent a phone number
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Use authentication via API Key
$config = Smscx\Client\Configuration::getDefaultConfiguration()->setApiKey('YOUR_API_KEY');
// OR
// Use authentication via Access Token
// $config = Smscx\Client\Configuration::getDefaultConfiguration()->setAccessToken('YOUR_ACCESS_TOKEN');
$smscx = new Smscx\Client\Api\NumbersApi(
new GuzzleHttp\Client(),
$config
);
$rent_number_request = [
'numberId' => '38d70eda-641c-4c1a-aae8-723ed8aef062',
'rentPeriod' => 30,
'autoRenew' => true,
'callbackUrl' => 'https://webhook-url/receive-inbound-sms',
];
try {
$result = $smscx->rentNumber($rent_number_request);
print_r($result);
// $result->getInfo()->getRentId();
// $result->getInfo()->getNumberId();
// $result->getInfo()->getPhoneNumber();
// $result->getInfo()->getCountryIso();
// $result->getInfo()->getRentStart();
// $result->getInfo()->getRentEnd();
// $result->getInfo()->getRentCost();
// $result->getInfo()->getSetupCost();
// $result->getInfo()->getAutoRenew();
// $result->getInfo()->getApproved();
// $result->getInfo()->getCallbackUrl();
// $result->getInfo()->getDatetime();
} catch (InvalidArgumentException $e) {
//Code for Invalid argument provided
} catch (Smscx\Client\Exception\InvalidRequestException $e) {
//Code for Invalid request
} catch (Smscx\Client\Exception\ResourceNotFoundException $e) {
//Number ID not found
} catch (Smscx\Client\Exception\AccessDeniedException $e) {
//Number was already rented by someone else
} catch (Smscx\Client\Exception\InsufficientBalanceException $e) {
//Code for Insufficient balance
} catch (Smscx\Client\Exception\ApiException $e) {
echo 'Exception when calling NumbersApi->rentNumber: ', $e->getMessage(), PHP_EOL;
}
Up to Table of Contents
Get inbound SMS
Manually check for the received SMS on a rented phone number. This method to get the list of received SMS is an alternative to the webhook method, in which the details of the inbound SMS are sent to a specified callback URL.
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Use authentication via API Key
$config = Smscx\Client\Configuration::getDefaultConfiguration()->setApiKey('YOUR_API_KEY');
// OR
// Use authentication via Access Token
// $config = Smscx\Client\Configuration::getDefaultConfiguration()->setAccessToken('YOUR_ACCESS_TOKEN');
$smscx = new Smscx\Client\Api\NumbersApi(
new GuzzleHttp\Client(),
$config
);
$rent_id = '471ddea7-930c-49e8-8e99-2683834dd92e';
try {
$result = $smscx->getInboundSms($rent_id);
print_r($result);
// $result->getInfo()->getRentId();
// $result->getInfo()->getPhoneNumber();
// $result->getInfo()->getCountryIso();
foreach ($result->getData() as $k => $v) {
// $v->getMsgId();
// $v->getFrom();
// $v->getCountryIso();
// $v->getTo();
// $v->getText();
// $v->getCost();
// $v->getReceivedAt();
}
} catch (InvalidArgumentException $e) {
//Code for Invalid argument provided
} catch (Smscx\Client\Exception\InvalidRequestException $e) {
//Code for Invalid request
} catch (Smscx\Client\Exception\ResourceNotFoundException $e) {
//Rent ID not found
} catch (Smscx\Client\Exception\ApiException $e) {
echo 'Exception when calling NumbersApi->getInboundSms: ', $e->getMessage(), PHP_EOL;
}
Up to Table of Contents
Get account balance
<?php
require_once(__DIR__ . '/vendor/autoload.php');
// Use authentication via API Key
$config = Smscx\Client\Configuration::getDefaultConfiguration()->setApiKey('YOUR_API_KEY');
// OR
// Use authentication via Access Token
// $config = Smscx\Client\Configuration::getDefaultConfiguration()->setAccessToken('YOUR_ACCESS_TOKEN');
$smscx = new Smscx\Client\Api\AccountApi(
new GuzzleHttp\Client(),
$config
);
try {
$result = $smscx->getAccountBalance();
print_r($result);
//$result->getBalance();
//$result->getCurrency();
//$result->getBilling();
} catch (Smscx\Client\ApiException $e) {
echo 'Exception when calling AccountApi->getAccountBalance: ', $e->getMessage(), PHP_EOL;
}
Up to Table of Contents