One-time PIN (OTP) SMS verification
A frequent method of verifying a user's phone number is by requesting the OTP (one-time password) delivered through SMS.
There are several ways to verify a phone number, but one of the most common is to send an SMS with a randomly generated one-time pin (OTP). Submitting this code to the company's website demonstrates ownership of the phone number.
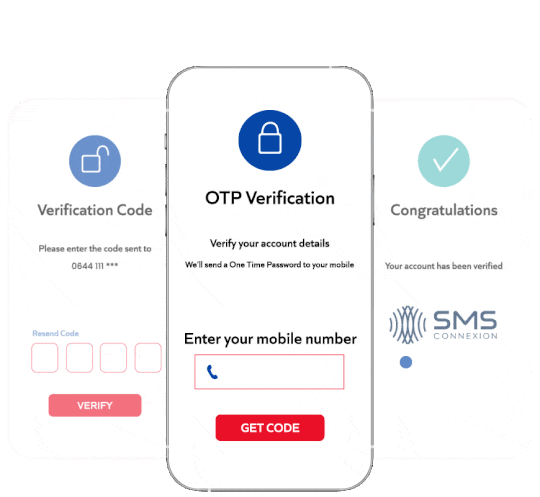
How to use OTP SMS verification
There are several ways that SMS OTP can be used
Phone number verification
Some services use a phone number as the main way to identify a user. In these services, users can prove who they are by entering their phone number and the one-time password (OTP) they get via SMS
Two-factor authentication
Together with the username and password, the SMS OTP (or SMS 2FA) can be a strong sign that the account belongs to the person who got the SMS OTP
Account restoration
If a person loses access to their account, they must be able to restore it. Sending an email to their registered address or SMS OTP to their phone is a frequent account recovery option
Payment confirmation
For security reasons, some banks or credit card companies ask the person making the payment for more proof of identity. OTP SMS is usually used for this purpose
Send OTP SMS with your own settings
Make sure your SMS OTP message is just how you want it. Customization options for one-time passwords (OTPs) let your recipients know who sent the SMS and let you set the security parameters for every generated password.
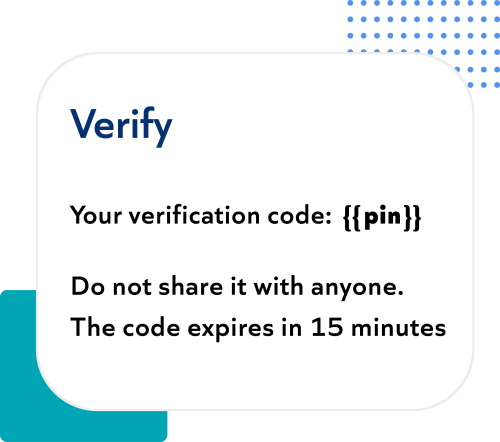
One Time Passwords through SMS
SMS is known for being reliable because 98% of people open it within 30 seconds. Sending one-time passwords through SMS ensures you reach your users wherever they are. Even when users don't have internet access, they can still use this OTP solution.
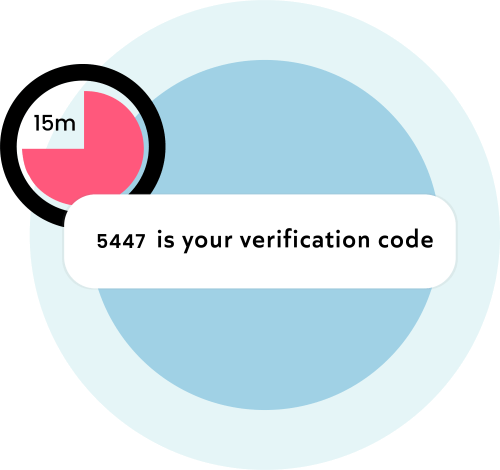
Code examples for OTP SMS API
Integrate our advanced OTP SMS API into your application and start verifying phone numbers in minutes.
Get up and running quickly with our official API wrappers and client libraries. They are available with popular languages like Python, PHP, Node.js, Java and others.
There's no client library for your language? Use a generic HTTP library of your choosing - it's quite simple.

curl --request POST \ --url https://api.sms.cx/otp \ --header 'Authorization: Bearer REPLACE_ACCESS_TOKEN' \ --header 'Content-Type: application/json' \ --data-raw '{ "phoneNumber": "+336124241xx", "from": "Verify", "template": "Your verification code is {{pin}}", "template_fr": "Votre code de vérification est {{pin}}", "template_de": "Ihr Bestätigungscode lautet {{pin}}", "template_es": "Tu código de verificación es {{pin}}", "template_it": "Il tuo codice di verifica è {{pin}}", "template_bg": "Вашият код за потвърждение е {{pin}}", "ttl": 600, "maxAttempts": 6, "pinType": "numbers", "pinLength": 5, "otpCallbackUrl": "https://my-callback/receive-otp-status" }'
import requests url = "https://api.sms.cx/otp" payload = { "phoneNumber": "+336124241xx", "from": "Verify", "template": "Your verification code is {{pin}}", "template_fr": "Votre code de vérification est {{pin}}", "template_de": "Ihr Bestätigungscode lautet {{pin}}", "template_es": "Tu código de verificación es {{pin}}", "template_it": "Il tuo codice di verifica è {{pin}}", "template_bg": "Вашият код за потвърждение е {{pin}}", "ttl": 300, "maxAttempts": 5, "pinType": "numbers", "pinLength": 5, "otpCallbackUrl": "https://my-callback/receive-otp-status" } headers = { "Content-Type": "application/json", "Authorization": "Bearer REPLACE_ACCESS_TOKEN" } response = requests.request("POST", url, json=payload, headers=headers) print(response.text)
require 'uri' require 'net/http' require 'openssl' url = URI("https://api.sms.cx/otp") http = Net::HTTP.new(url.host, url.port) http.use_ssl = true http.verify_mode = OpenSSL::SSL::VERIFY_NONE request = Net::HTTP::Post.new(url) request["Content-Type"] = 'application/json' request["Authorization"] = 'Bearer REPLACE_ACCESS_TOKEN' request.body = '{"phoneNumber":"+336124241xx","from":"Verify","template":"Your verification code is {{pin}}","template_fr":"Votre code de vérification est {{pin}}","template_de":"Ihr Bestätigungscode lautet {{pin}}","template_es":"Tu código de verificación es {{pin}}","template_it":"Il tuo codice di verifica è {{pin}}","template_bg":"Вашият код за потвърждение е {{pin}}","ttl":600,"maxAttempts":6,"pinType":"numbers","pinLength":5,"otpCallbackUrl":"https://my-callback/receive-otp-status"}' response = http.request(request) puts response.read_body
const data = JSON.stringify({ "phoneNumber": "+336124241xx", "from": "Verify", "template": "Your verification code is {{pin}}", "template_fr": "Votre code de vérification est {{pin}}", "template_de": "Ihr Bestätigungscode lautet {{pin}}", "template_es": "Tu código de verificación es {{pin}}", "template_it": "Il tuo codice di verifica è {{pin}}", "template_bg": "Вашият код за потвърждение е {{pin}}", "ttl": 300, "maxAttempts": 5, "pinType": "numbers", "pinLength": 5, "otpCallbackUrl": "https://my-callback/receive-otp-status" }); const xhr = new XMLHttpRequest(); xhr.withCredentials = true; xhr.addEventListener("readystatechange", function () { if (this.readyState === this.DONE) { console.log(this.responseText); } }); xhr.open("POST", "https://api.sms.cx/otp"); xhr.setRequestHeader("Content-Type", "application/json"); xhr.setRequestHeader("Authorization", "Bearer REPLACE_ACCESS_TOKEN"); xhr.send(data);
const http = require("https"); const options = { "method": "POST", "hostname": "api.sms.cx", "port": null, "path": "/otp", "headers": { "Content-Type": "application/json", "Authorization": "Bearer REPLACE_ACCESS_TOKEN" } }; const req = http.request(options, function (res) { const chunks = []; res.on("data", function (chunk) { chunks.push(chunk); }); res.on("end", function () { const body = Buffer.concat(chunks); console.log(body.toString()); }); }); req.write(JSON.stringify({ phoneNumber: '+336124241xx', from: 'Verify', template: 'Your verification code is {{pin}}', template_fr: 'Votre code de vérification est {{pin}}"', template_de: 'Ihr Bestätigungscode lautet {{pin}}', template_es: 'Tu código de verificación es {{pin}}', template_it: 'Il tuo codice di verifica è {{pin}}', template_bg: 'Вашият код за потвърждение е {{pin}}', ttl: 300, maxAttempts: 5, pinType: 'numbers', pinLength: 5, otpCallbackUrl: 'https://my-callback/receive-otp-status' })); req.end();
<?php $curl = curl_init(); $payload = [ "phoneNumber" => "+336124241xx", "from" => "Verify", "template" => "Your verification code is {{pin}}", "template_fr" => "Votre code de vérification est {{pin}}", "template_de" => "Ihr Bestätigungscode lautet {{pin}}", "template_es" => "Tu código de verificación es {{pin}}", "template_it" => "Il tuo codice di verifica è {{pin}}", "template_bg" => "Вашият код за потвърждение е {{pin}}", "ttl" => 600, "maxAttempts" => 6, "pinType" => "numbers", "pinLength" => 5, "otpCallbackUrl" => "https://my-callback/receive-otp-status", ]; curl_setopt_array($curl, [ CURLOPT_URL => "https://api.sms.cx/otp", CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => "", CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 30, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => "POST", CURLOPT_POSTFIELDS => json_encode($payload), CURLOPT_HTTPHEADER => [ "Authorization: Bearer REPLACE_ACCESS_TOKEN", "Content-Type: application/json" ], ]); $response = curl_exec($curl); $err = curl_error($curl); curl_close($curl); if ($err) { echo "cURL Error #:" . $err; } else { echo $response; }
OkHttpClient client = new OkHttpClient(); MediaType mediaType = MediaType.parse("application/json"); RequestBody body = RequestBody.create(mediaType, "{\"phoneNumber\":\"+336124241xx\",\"from\":\"Verify\",\"template\":\"Your verification code is {{pin}}\",\"template_fr\":\"Votre code de v\u00E9rification est {{pin}}\",\"template_de\":\"Ihr Best\u00E4tigungscode lautet {{pin}}\",\"template_es\":\"Tu c\u00F3digo de verificaci\u00F3n es {{pin}}\",\"template_it\":\"Il tuo codice di verifica \u00E8 {{pin}}\",\"template_bg\":\"\u0412\u0430\u0448\u0438\u044F\u0442 \u043A\u043E\u0434 \u0437\u0430 \u043F\u043E\u0442\u0432\u044A\u0440\u0436\u0434\u0435\u043D\u0438\u0435 \u0435 {{pin}}\",\"ttl\":600,\"maxAttempts\":6,\"pinType\":\"numbers\",\"pinLength\":5,\"otpCallbackUrl\":\"https:\/\/my-callback\/receive-otp-status\"}"); Request request = new Request.Builder() .url("https://api.sms.cx/otp") .post(body) .addHeader("Content-Type", "application/json") .addHeader("Authorization", "Bearer REPLACE_ACCESS_TOKEN") .build(); Response response = client.newCall(request).execute();
var client = new RestClient("https://api.sms.cx/otp"); var request = new RestRequest(Method.POST); request.AddHeader("Content-Type", "application/json"); request.AddHeader("Authorization", "Bearer REPLACE_ACCESS_TOKEN"); request.AddParameter("application/json", "{\"phoneNumber\":\"+336124241xx\",\"from\":\"Verify\",\"template\":\"Your verification code is {{pin}}\",\"template_fr\":\"Votre code de v\u00E9rification est {{pin}}\",\"template_de\":\"Ihr Best\u00E4tigungscode lautet {{pin}}\",\"template_es\":\"Tu c\u00F3digo de verificaci\u00F3n es {{pin}}\",\"template_it\":\"Il tuo codice di verifica \u00E8 {{pin}}\",\"template_bg\":\"\u0412\u0430\u0448\u0438\u044F\u0442 \u043A\u043E\u0434 \u0437\u0430 \u043F\u043E\u0442\u0432\u044A\u0440\u0436\u0434\u0435\u043D\u0438\u0435 \u0435 {{pin}}\",\"ttl\":600,\"maxAttempts\":6,\"pinType\":\"numbers\",\"pinLength\":5,\"otpCallbackUrl\":\"https:\/\/my-callback\/receive-otp-status\"}", ParameterType.RequestBody); IRestResponse response = client.Execute(request);
package main import ( "fmt" "strings" "net/http" "io/ioutil" ) func main() { url := "https://api.sms.cx/otp" payload := strings.NewReader("{\"phoneNumber\":\"+336124241xx\",\"from\":\"Verify\",\"template\":\"Your verification code is {{pin}}\",\"template_fr\":\"Votre code de v\u00E9rification est {{pin}}\",\"template_de\":\"Ihr Best\u00E4tigungscode lautet {{pin}}\",\"template_es\":\"Tu c\u00F3digo de verificaci\u00F3n es {{pin}}\",\"template_it\":\"Il tuo codice di verifica \u00E8 {{pin}}\",\"template_bg\":\"\u0412\u0430\u0448\u0438\u044F\u0442 \u043A\u043E\u0434 \u0437\u0430 \u043F\u043E\u0442\u0432\u044A\u0440\u0436\u0434\u0435\u043D\u0438\u0435 \u0435 {{pin}}\",\"ttl\":600,\"maxAttempts\":6,\"pinType\":\"numbers\",\"pinLength\":5,\"otpCallbackUrl\":\"https:\/\/my-callback\/receive-otp-status\"}") req, _ := http.NewRequest("POST", url, payload) req.Header.Add("Content-Type", "application/json") req.Header.Add("Authorization", "Bearer REPLACE_ACCESS_TOKEN") res, _ := http.DefaultClient.Do(req) defer res.Body.Close() body, _ := ioutil.ReadAll(res.Body) fmt.Println(res) fmt.Println(string(body)) }