Phone number lookup
Phone number lookup (or HLR lookup) allows for accurate and up-to-date information about a phone number, such as current status (active or absent from network), or if the number has been ported to another carrier or if it is currently roaming on a different network outside the country.
Keep your database clean by regularly removing outdated or inaccurate phone numbers to ensure that messages and calls are only sent to valid, active contacts. This helps to optimize costs by reducing the number of failed or undelivered SMS messages and calls.
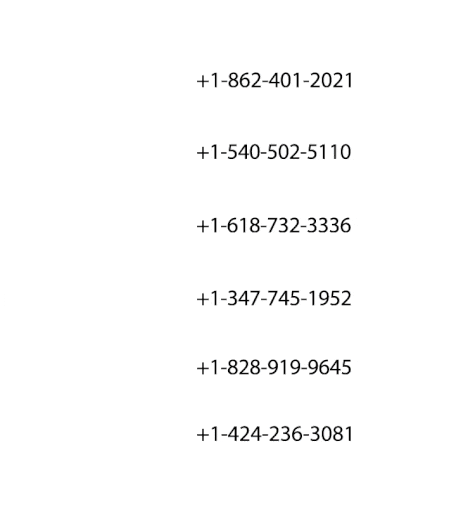
Phone number lookup features
There are several features of phone number carrier lookup from which we highlight:
Global coverage
More than 220 countries are currently supported by the SMS.CX Phone Number Lookup service. Our international phone numbers lookup service can let your business engage no matter where it is
Real-time lookup
Use precise and reliable number details generated by phone number lookup, which includes accurate information about portability and roaming in real time
Bulk phone lookup
Use our interactive web platform and API to start bulk phone numbers lookup campaigns in minutes. Reduce messaging costs by using a phone number validator
What details you get from a phone number lookup
Phone number carrier lookup is a way to check if a phone number is valid and also checks for the active status and network availability of a mobile number.
A phone number lookup can provide various details. Some of the most important details you can obtain from a phone carrier lookup are:
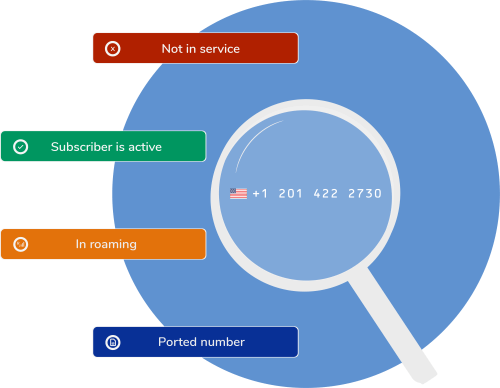
Use our Web Platform for bulk number lookup campaigns
Our easy-to-use interface allows you to easily upload large lists of phone numbers for a fast and efficient processing of bulk phone number carrier lookup.
It is possible to view reports for number lookup campaigns and export them in different formats.
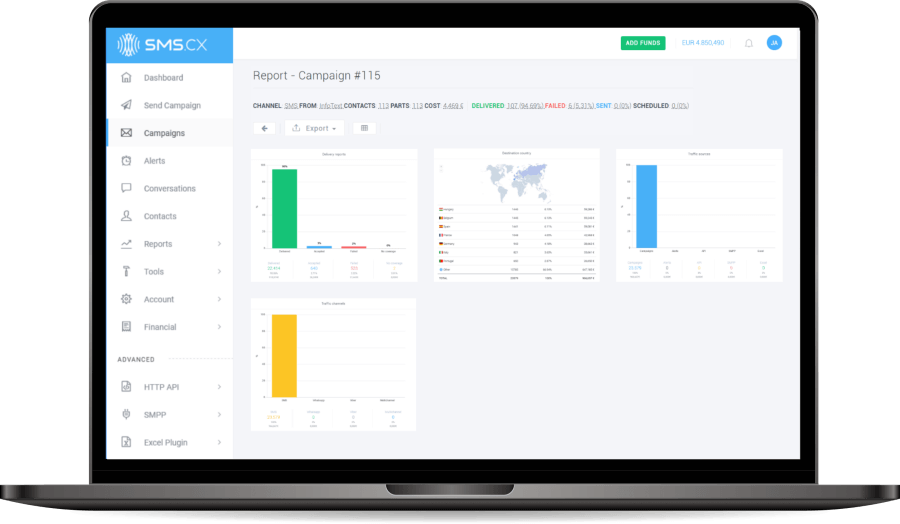
Code examples for Phone Number Lookup API
Integrate our advanced Phone Lookup API into your application and start phone validation in minutes.
Make requests to the API to perform bulk phone number lookup (up to 40.000 phone numbers can be validated in one single API call).
Get started in no time with our official API wrappers and client libraries. They are available in popular languages like Python, PHP, Node.js, Java, and others.
There is no client library for your preferred language? You can use any HTTP library, it's easy.
curl --request POST \
--url https://api.sms.cx/numbers/lookup \
--header 'Authorization: Bearer REPLACE_ACCESS_TOKEN' \
--header 'Content-Type: application/json' \
--data-raw '{
"phoneNumbers": [
"+336129353",
"+33612970283",
"+3361211",
"+43664187834",
"+41781218472",
"+351912110421",
"+4915123473140",
"+4915123595",
"+4915123966046"
]
}'
import requests
url = "https://api.sms.cx/numbers/lookup"
payload = {
"phoneNumbers": [
"+336129353",
"+33612970283",
"+3361211",
"+43664187834",
"+41781218472",
"+351912110421",
"+4915123473140",
"+4915123595",
"+4915123966046"
]
}
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer REPLACE_ACCESS_TOKEN"
}
response = requests.request("POST", url, json=payload, headers=headers)
print(response.text)
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://api.sms.cx/numbers/lookup")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["Content-Type"] = 'application/json'
request["Authorization"] = 'Bearer REPLACE_ACCESS_TOKEN'
request.body = "{\"phoneNumbers\":[\"+336129353\",\"+33612970283\",\"+3361211\",\"+43664187834\",\"+41781218472\",\"+351912110421\",\"+4915123473140\",\"+4915123595\",\"+4915123966046\"]}"
response = http.request(request)
puts response.read_body
<?php
$curl = curl_init();
$payload = [
"phoneNumbers" => [
"+336129353",
"+33612970283",
"+3361211",
"+43664187834",
"+41781218472",
"+351912110421",
"+4915123473140",
"+4915123595",
"+4915123966046",
]
];
curl_setopt_array($curl, [
CURLOPT_URL => "https://api.sms.cx/numbers/lookup",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => json_encode($payload),
CURLOPT_HTTPHEADER => [
"Authorization: Bearer REPLACE_ACCESS_TOKEN",
"Content-Type: application/json"
],
]);
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
OkHttpClient client = new OkHttpClient();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\"phoneNumbers\":[\"+336129353\",\"+33612970283\",\"+3361211\",\"+43664187834\",\"+41781218472\",\"+351912110421\",\"+4915123473140\",\"+4915123595\",\"+4915123966046\"]}");
Request request = new Request.Builder()
.url("https://api.sms.cx/numbers/lookup")
.post(body)
.addHeader("Content-Type", "application/json")
.addHeader("Authorization", "Bearer REPLACE_ACCESS_TOKEN")
.build();
Response response = client.newCall(request).execute();
const http = require("https");
const options = {
"method": "POST",
"hostname": "api.sms.cx",
"port": null,
"path": "/numbers/lookup",
"headers": {
"Content-Type": "application/json",
"Authorization": "Bearer REPLACE_ACCESS_TOKEN"
}
};
const req = http.request(options, function (res) {
const chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function () {
const body = Buffer.concat(chunks);
console.log(body.toString());
});
});
req.write(JSON.stringify({
phoneNumbers: [
'+336129353',
'+33612970283',
'+3361211',
'+43664187834',
'+41781218472',
'+351912110421',
'+4915123473140',
'+4915123595',
'+4915123966046'
]
}));
req.end();
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.sms.cx/numbers/lookup"
payload := strings.NewReader("{\"phoneNumbers\":[\"+336129353\",\"+33612970283\",\"+3361211\",\"+43664187834\",\"+41781218472\",\"+351912110421\",\"+4915123473140\",\"+4915123595\",\"+4915123966046\"]}")
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("Content-Type", "application/json")
req.Header.Add("Authorization", "Bearer REPLACE_ACCESS_TOKEN")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := ioutil.ReadAll(res.Body)
fmt.Println(res)
fmt.Println(string(body))
}
var client = new RestClient("https://api.sms.cx/numbers/lookup");
var request = new RestRequest(Method.POST);
request.AddHeader("Content-Type", "application/json");
request.AddHeader("Authorization", "Bearer REPLACE_ACCESS_TOKEN");
request.AddParameter("application/json", "{\"phoneNumbers\":[\"+336129353\",\"+33612970283\",\"+3361211\",\"+43664187834\",\"+41781218472\",\"+351912110421\",\"+4915123473140\",\"+4915123595\",\"+4915123966046\"]}", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
How to make bulk phone lookup from SMS.CX online platform
Features comparison between phone number services
There are several ways to check if a phone number is valid. One method is to use a phone number validation tool, which we provide free of charge. This phone validator tool use algorithms and rules to verify the format and structure of the phone number, ensuring that it follows the correct formatting for the country or region.
Phone number lookup, on the other hand, is a process used to validate a mobile phone number by checking it against a database of registered mobile numbers and their associated information, such as network provider and also making a phone number portability check. This helps to ensure that the number is not only valid, but also currently in use and reachable on the relevant mobile network.
In summary, phone number validation checks for the correctness and format of a phone number, while phone number lookup checks for the active status and network availability of a mobile number.
We provide different services for phone numbers: validation, lookup (also called as HLR lookup) and verify (also called as OTP SMS).
Below is a table with feature comparison and cost for each of these services.
What is HLR lookup?
HLR lookup, or Home Location Register Lookup, is a database that stores information about mobile phone numbers and its associated subscribers. Our phone carrier lookup service accesses this database to retrieve information about a particular number, including its current status.
HLR carrier lookup can check for the portability status and roaming of a phone number. This is a common feature of phone validation service, which use HLR lookup technology to verify the accuracy and validity of a phone number. The phone number carrier lookup can determine whether a number is active, whether it is currently roaming on another network, and whether it has been recently ported to a different carrier. This information is important for businesses and organizations that rely on accurate and up-to-date phone numbers for customer communication and outreach.
The HLR Lookup API checks a mobile phone number's status by querying a mobile network operator's database. This request is sent via API, which is secure and fast. The HLR Lookup API returns a response once the request is received by the mobile network operator's database. This response can include information like whether the number is active or not, whether it is roaming, and whether it is a valid number.
What are the benefits of using phone carrier lookup?
The HLR Lookup API is accurate and reliable. Because the API is connected to the mobile network operator's database, it will offer real-time mobile phone number status. Businesses can use the API's metadata to decide how to handle calls or messages to a specific number.
HLR Lookup API is fast and efficient. Businesses may check a large number of mobile phone numbers rapidly using the phone number lookup API. This is helpful for companies who need to verify a big number of mobile phones for a marketing campaign or other activity.
The HLR Lookup API and Web Platform are useful tools for companies who need to verify mobile phone numbers. The API's accuracy, reliability, and speed can help businesses make more intelligent decisions about how to handle calls and messages to specific numbers and can give information about the countries or network operators associated with those phone numbers.
What is phone number portability?
Phone number portability, also known as number porting, is the ability for a customer to retain their phone number when switching to a new provider. This is a convenient option for people who don't want to update their contacts with a new phone number or who want to keep their current phone number for professional or personal reasons.
For example, John wants to switch providers because he's unhappy with his present one. With phone number portability, he can keep his present number and switch providers. All of his contacts will still be able to reach him at the same number, and he won't have to update everyone.
What is phone number roaming?
Phone number roaming allows users to make and receive calls, send and receive text messages, and access data services outside their home network's coverage region. Mobile phone networks have agreements with other networks to let consumers access their services while roaming.
When roaming, a user's phone number stays the same, but their network may vary. A roaming mobile phone number means the user is outside of their home network and utilizing another to make and receive calls or access data services. This happens when a person is traveling and their home network isn't available. Our phone number lookup service allows you to determine whether a particular mobile phone number is roaming or not.
Why should you use phone number lookup regularly?
It is important to note that phone numbers can become invalid over time. For example, if a person changes their phone number or a business closes down, their old phone number may no longer be in use. In these cases, it is important to check for updates and verify that the phone number is still valid.
Overall, verifying the validity of a phone number is crucial for successful communication. By using a phone validator tool with carrier lookup capability, you can ensure that your database is always clean and you send SMS campaigns only to active subscribers.
How to start with Phone Number Lookup
On this page, we went into detail about why you should use the phone number carrier lookup service. Here are some next steps:
- Signup for an account to perform number carrier lookup for phone number validation
- View examples of Phone Numbers Lookup API methods (see bulk lookup campaigns, process bulk phone number lookup, make single phone number lookup) with our SDK and API client libraries
- Read the full documentation about how to use the Phone Numbers Lookup API